算法和理解能力太差了,做题感觉自己像脑缠,先从古典密码入手
参考
https://zhuanlan.zhihu.com/p/222691227
凯撒密码
凯撒加密就是将明文中的每个字母都按照其在字母表中的顺序向后或向前移动固定数目(循环移动)作为密文,这个固定数目就是key
下面给出encode和decode的实现(我这里还添加了对数字的Caesar)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
| #define _CRT_SECURE_NO_WARNINGS #include<stdio.h> #include<string.h> #include<Windows.h>
void Caesar_encode(char raw[], int key) { int i; for (i = 0; i < strlen(raw); ++i) { if (raw[i] >= 'A' && raw[i] <= 'Z') { raw[i] = (raw[i] - 'A' + key) % 26 + 'A'; } else if (raw[i] >= 'a' && raw[i] <= 'z') { raw[i]= (raw[i] - 'a' + key) % 26 + 'a'; } else if (raw[i] >= '0' && raw[i] <= '9') { raw[i] = (raw[i] - '0' + key) % 10 + '0'; } } printf("加密后的字符串为:%s\n", raw); }
void Caesar_decode(char raw[], int key) { int i; for (i = 0; i < strlen(raw); ++i) { if (raw[i] >= 'A' && raw[i] <= 'Z') { raw[i] = (raw[i] - 'A' + 26 - key) % 26 + 'A'; } else if (raw[i] >= 'a' && raw[i] <= 'z') { raw[i] = (raw[i] - 'a' + 26 - key) % 26 + 'a'; } else if (raw[i] >= '0' && raw[i] <= '9') { raw[i] = (raw[i] - '0' + 10 - key) % 10 + '0'; } } printf("解密后的字符串为:%s\n", raw); return; } int main() { int key = 0; char raw[] = "flag123123asdasdasdzxczaqw";
printf("Please input your key\n"); scanf("%d", &key); char encode[] = {0}; char decode[] = {0}; Caesar_encode(raw, key); Caesar_decode(raw, key); return 0; }
|
基于密钥的Caesar
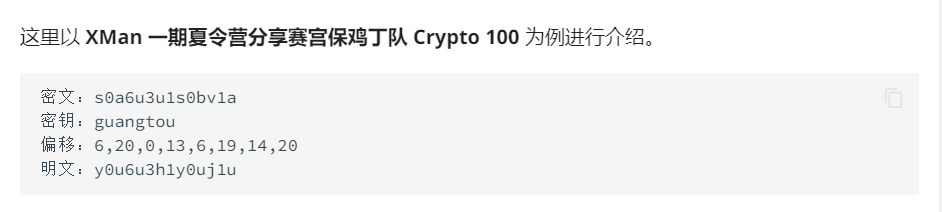
先找到密钥各个字母在字母表中的偏移,然后以此偏移作为密钥加密原文中的字母
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| void Caesar_key_encode(char* raw, char* key_str) { int j = 0; for (int i = 0; i < strlen(raw); ++i) { if (raw[i] >= 'A' && raw[i] <= 'Z') { raw[i] = (raw[i] - 'A' + key_str[j] - 'a') % 26 + 'A'; j++; } else if (raw[i] >= 'a' && raw[i] <= 'z') { raw[i] = (raw[i] - 'a' + key_str[j] - 'a') % 26 + 'a'; j++; } } printf("密钥凯撒加密后的结果:%s\n", raw); return; }
|
仿射变换